728x90
반응형
문제 출처 : https://www.acmicpc.net/problem/14938
14938번: 서강그라운드
예은이는 요즘 가장 인기가 있는 게임 서강그라운드를 즐기고 있다. 서강그라운드는 여러 지역중 하나의 지역에 낙하산을 타고 낙하하여, 그 지역에 떨어져 있는 아이템들을 이용해 서바이벌을
www.acmicpc.net
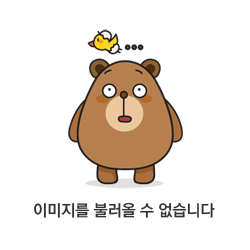
풀이
다익스트라로 해결하는 문제이다. 최대 아이템 개수를 구해야 하므로, 모든 지역별로 다익스트라를 돌린 후, 최대 아이템을 갱신하면 된다.
다익스트라
우선순위 큐를 이용해서 구현하였다.
void dijkstra(int start) {
for(int i = 1; i <= n; i++)
dist[i] = INF;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, start});
dist[start] = 0;
while(!pq.empty()) {
int cost = pq.top().first;
int cur = pq.top().second;
pq.pop();
for(int i = 0; i < edge[cur].size(); i++) {
int next = edge[cur][i].first;
int ncost = edge[cur][i].second;
if(dist[next] > cost + ncost) {
dist[next] = cost + ncost;
pq.push({dist[next], next});
}
}
}
}
코드
#include <bits/stdc++.h>
using namespace std;
#define INF 987654321
int n, m, r;
int ans;
int dist[101];
vector<pair<int, int>> edge[101];
void find_item(int start, vector<int> items) {
int sum = 0;
for(int i = 1; i <= n; i++) {
if(dist[i] <= m) {
sum += items[i];
}
}
ans = max(ans, sum);
}
void dijkstra(int start) {
for(int i = 1; i <= n; i++)
dist[i] = INF;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, start});
dist[start] = 0;
while(!pq.empty()) {
int cost = pq.top().first;
int cur = pq.top().second;
pq.pop();
for(int i = 0; i < edge[cur].size(); i++) {
int next = edge[cur][i].first;
int ncost = edge[cur][i].second;
if(dist[next] > cost + ncost) {
dist[next] = cost + ncost;
pq.push({dist[next], next});
}
}
}
}
int main(void){
ios::sync_with_stdio(false); cin.tie(NULL); cout.tie(NULL);
cin >> n >> m >> r;
vector<int> items(n+1);
for(int i = 1; i <= n; i++) {
cin >> items[i];
}
for(int i = 0; i < r; i++) {
int a, b, l;
cin >> a >> b >> l;
edge[a].push_back({b, l});
edge[b].push_back({a, l});
}
for(int i = 1; i <= n; i++) {
dijkstra(i);
find_item(i, items);
}
cout << ans << '\n';
return 0;
}
728x90
반응형
'Tech > Algorithm' 카테고리의 다른 글
[BOJ] 백준 2146 다리 만들기 c++ (dfs, bfs) (0) | 2023.10.20 |
---|---|
[BOJ] 백준 2636 치즈 c++ (bfs) (2) | 2023.10.11 |
[프로그래머스] 디펜스 게임 c++ (우선순위 큐) (0) | 2023.09.12 |
[프로그래머스] 미로 탈출 c++ (bfs) (0) | 2023.09.08 |
[BOJ] 백준 14728 벼락치기 c++ (배낭, dp) (0) | 2023.08.01 |